Back just a few years ago it was required for web developers use JavaScript/jQuery to perform animated effects in-browser. CSS3 has dramatically changed the rules of the game where you can animate any standard property of an HTML element. This opens up a whole new room of effects you can put together in just 15-20 minutes of tinkering with code. My example below uses a few Dribbble shots as a demo of how you can setup animated box effects. The style appears when you hover over each image to display some further information such as the title, description, and publication date. I’ll be explaining how to build a similar effect on your own website using nothing but HTML5 and CSS3 techniques. ![]() Live Demo – Download Source Code Browser SupportBefore we begin I should bring up a small tidbit about browser support.Unfortunately only the latest -and-greatest rendering engines will utilize these CSS3 animation properties. The most notable browsers to support these features include Apple’s Safari, Mozilla Firefox 4+, Google Chrome, Opera, and as of the latest release Internet Explorer 9+. None of the earlier IE versions 6-8 have native support for these properties. But there are hack-ish workarounds you can implement to get them up and running. Structuring the HTMLI’m using a very minimalist layout to demonstrate how easy it is to implement these effects.The main document is wrapped in a #container div which is centered on the page 600px wide.Then each inner box is given the class .boxxy which uses box-shadows and a slight transitioneffect on hover. <div id="container"> <div class="boxxy"> <a href="http://dribbble.com/shots/489532-Banking-App" target="_blank" class="anchor-hover"> <img src="images/sendmoney.png" width="400" height="300" /> <span class="details"> <h2>Banking App</h2> <p class="desc">Here's a full shot of the send money transaction for this "secret" banking app which I've been working on. This is an early concept, I haven't landed the full project yet, but hoping they like the concept so far.</p> <span class="pubdate">Published March 28, 2012</span> <span class="viewlink">View on Dribbble →</span> </span> </a> </div>Possibly the most interesting piece of this code is our span classed .details .The opacity for this block is originally set to 0 so we can’t see any of the content. However, once you hover over the anchor link it targets the inner details box and animates to 100% opacity. I’ve set the background of this detail box to 85% so that you can still see through to the original image shot. This can be accomplished using the CSS rgba() function which is also supported in all mainstream browsers. Now let’s check out some of these cool CSS effects in action. Box Shadow DesignI’m going to start on the pink box shadow animation first. This is calledwhenever you hover over the outer .boxxy element, but it still holds true when hovering over the image as well. .boxxy { display: block; margin: 0 auto; background: #fff; margin-bottom: 22px; -webkit-box-shadow: 0 2px 3px rgba(0, 0, 0, 0.2); -moz-box-shadow: 0 2px 3px rgba(0, 0, 0, 0.2); box-shadow: 0 2px 3px rgba(0, 0, 0, 0.2); width: 400px; padding: 7px 9px; transition: box-shadow 0.3s linear 0s; -webkit-transition: box-shadow 0.3s linear 0s; -moz-transition: box-shadow 0.3s linear 0s; -o-transition: box-shadow 0.3s linear 0s; }The code above are styles for the boxxy class. Notice that we have to add each -webkit-box-shadow ,-moz-box-shadow , and box-shadow properties for full browser support.Also after these properties you’ll find each of the different transition methods. It’s important that these are added on the original element and not the hover selector. The way you perform animations is by changing different properties on a user action(hover/focus) – such as font color or box shadows. Then you add the animation cues to the original style selector and it will transition gracefully from the normal state into the hover state and back again. This can be explained by looking at one example from our transition properties. transition: box-shadow 0.3s linear 0s;The single line above is copied out of our .boxxy animations for the pink box shadow. This tells CSS to animate only our box-shadow property. We want the animation to last 0.3 seconds using the linear effect. This “effect” is also known as our transition timing function. The final piece of this transition property is for a possible animation delay, which I’ve used 0 seconds. Check out this page on CSS3 transitions syntax to get a better idea of how it all works. Looking at Hover StatesI’m going to add in the code for both our .boxxy hover and the details hover effect. Remember that neither of these selectors need to have the transition properties, as they are already declared on the normal class selector. .anchor-hover:hover .details { opacity: 1; } .boxxy:hover { box-shadow: 0 1px 2px rgba(0, 0, 0, 0.15) inset, 0 0 10px rgba(182, 70, 165, 0.7); -moz-box-shadow: 0 1px 2px rgba(0, 0, 0, 0.15) inset, 0 0 10px rgba(182, 70, 165, 0.7); -webkit-box-shadow: 0 1px 2px rgba(0, 0, 0, 0.15) inset, 0 0 10px rgba(182, 70, 165, 0.7); }The boxxy code is using two different CSS box-shadow effects to create an outer glow and an inset shadow. Of course, the details pane is a lot simpler and only needs to be animated into a higher opacity. You can try implementing other changes and include them in our original transitions for added effects. Styling the Shot DetailsNow last but not least we’ll take a look at all the CSS styles for buildingthe internal details pane. This container is home to a number of smaller elements including a header, paragraph description, and live published date. .anchor-hover .details { opacity: 0; position: absolute; top: 0px; left: 0px; width: 390px; height: 290px; margin: 0; padding-top: 10px; padding-left: 10px; font-size: 1.2em; line-height: 1.4em; text-decoration: none; color:black; background: rgba(255, 255, 255, 0.85); overflow: hidden; transition: opacity 0.25s linear 0s; -webkit-transition: opacity 0.25s linear 0s; -moz-transition: opacity 0.25s linear 0s; -o-transition: opacity 0.25s linear 0s; } .anchor-hover .details h2 { font-weight: bold; font-size: 1.5em; color: black; text-decoration: none; margin-bottom: 8px; } .anchor-hover .details p.desc { font-weight: normal; font-size: 1.2em; line-height: 1.3em; color: black; } .anchor-hover .details span.pubdate { position: absolute; bottom: 10px; left: 10px; font-weight: bold; font-family: "Trebuchet MS", Tahoma, sans-serif; } .anchor-hover .details span.viewlink { position: absolute; bottom: 10px; right: 10px; font-weight: bold; color: black; font-size: 1.3em; }The .details box is using absolute positioning within the original container.I’ve removed 10px from the width and height so the extra white background doesn’t overlap outside the box(I’m also using overflow: hidden; to counteract this). Again we have the four different transitionproperties for standard CSS, WebKit Engines, Firefox/Gecko, and Opera. This time the animations are targeting the opacity property with a 25 millisecond animation. It’s all fairly straightforward and easy to understand if you’ve been using CSS for a while. I’ll admit that transitions can be confusing because of the largely cryptic syntax. But the best way to memorize these properties is through repetition and practice in your own work! ![]() Live Demo – Download Source Code ConclusionIt is hope this tutorial acts as a powerful introduction to the world of CSS3 animations.I’ve only covered a couple of different effects you can use. But with the versatile nature of CSS properties it’s so incredibly easy to customize your own animations for practically any HTML elements. Be sure to check out our live demo example above. And if you want to mess around with code feel free to download the .zip archive as well. |
MyBLog
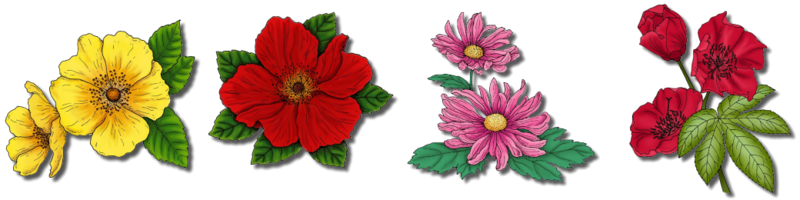
Tuesday, April 3, 2012
Using CSS3 Animations to Build a Sleek Box UI
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment